1.9. Bookmarkable URLs with the Blog example
Seam makes it easy to implement applications which keep state on the server side. However, server-side state is not always appropriate, particularly for functionality that serves up content. For this, application state is often stored as part of the URL, so that any page can be accessed through a bookmark at any time. The blog example shows how to implement an application that supports bookmarking throughout, even on the search results page. This example demonstrates Seam's management of application state in the URL.
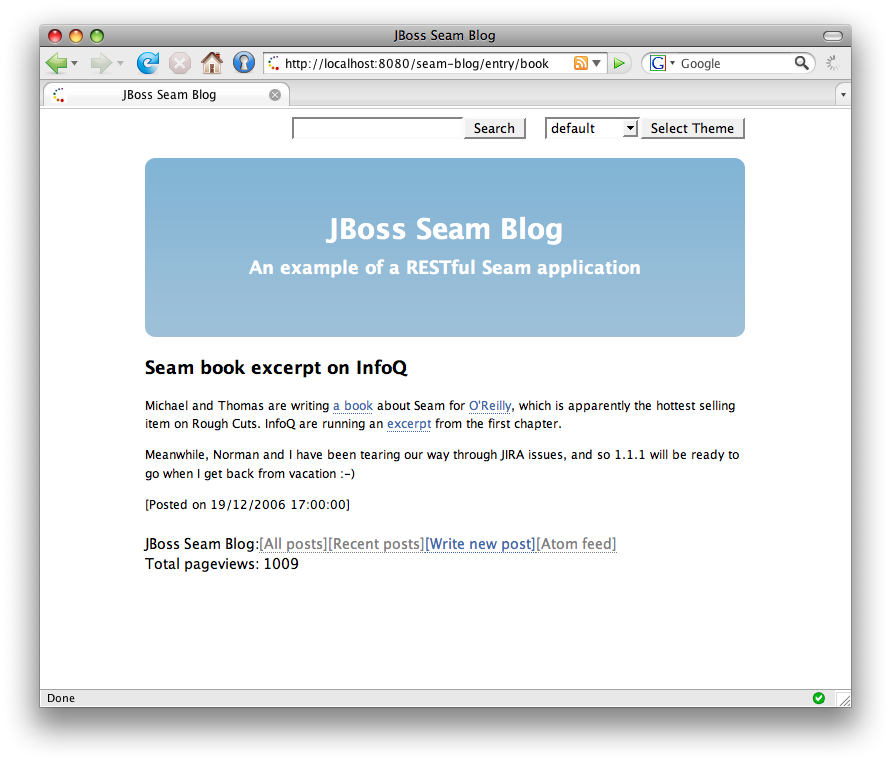
The blog example demonstrates the use of "pull"-style model view control (MVC), where the view pulls data from components as it is being rendered rather than using action listener methods to retrieve and prepare data for the view.
1.9.1. Using "pull"-style MVC
This snippet from the
index.xhtml
facelets page displays a list of recent blog entries:
<h:dataTable value="#{blog.recentBlogEntries}" var="blogEntry" rows="3"> <h:column> <div class="blogEntry"> <h3>#{blogEntry.title}</h3> <div> <s:formattedText value="#{blogEntry.excerpt==null ? blogEntry.body : blogEntry.excerpt}"/> </div> <p> <s:link view="/entry.xhtml" rendered="#{blogEntry.excerpt!=null}" propagation="none" value="Read more..."> <f:param name="blogEntryId" value="#{blogEntry.id}"/> </s:link> </p> <p> [Posted on  <h:outputText value="#{blogEntry.date}"> <f:convertDateTime timeZone="#{blog.timeZone}" locale="#{blog.locale}" type="both"/> </h:outputText>]   <s:link view="/entry.xhtml" propagation="none" value="[Link]"> <f:param name="blogEntryId" value="#{blogEntry.id}"/> </s:link> </p> </div> </h:column> </h:dataTable>
If we navigate to this page from a bookmark, the
#{blog.recentBlogEntries}
data used by the <h:dataTable>
is retrieved lazily — "pulled" — when required, by a Seam component named blog
. This flow of control is the reverse of that used in traditional action-based web frameworks like Struts.
Example 1.34.
@Name("blog") @Scope(ScopeType.STATELESS) @AutoCreate public class BlogService { @In EntityManager entityManager;@Unwrap
public Blog getBlog() { return (Blog) entityManager.createQuery("select distinct b from Blog b left join fetch b.blogEntries") .setHint("org.hibernate.cacheable", true) .getSingleResult(); } }
This component uses a seam-managed persistence context. Unlike the other examples we've seen, this persistence context is managed by Seam, instead of by the EJB3 container. The persistence context spans the entire web request, allowing us to avoid any exceptions that occur when accessing unfetched associations in the view.
| |
The @Unwrap annotation tells Seam to provide the return value of the method — the Blog — instead of the actual BlogService component to clients. This is the Seam manager component pattern.
|
This will store basic view content, but to bookmark form submission results like a search results page, there are several other required definitions.