Red Hat Training
A Red Hat training course is available for JBoss Enterprise SOA Platform
Chapter 15. Test Driven Development for Workflow
15.1. Introducing Test Driven Development for Workflow
Read this chapter to learn how to use JUnit without any extensions to unit test custom process definitions.
Keep the development cycle as short as possible. Verify all changes to software source code immediately, (preferably, without any intermediate build steps.) The following examples demonstrate how to develop and test jBPM processes in this way.
Most process definition unit tests are execution-based. Each scenario is executed in one JUnit test method and this feeds the external triggers (signals) into a process execution. It then verifies after each signal to confirm that the process is in the expected state.
Here is an example graphical representation of such a test. It takes a simplified version of the auction process:
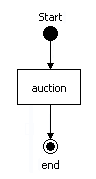
Figure 15.1. The auction test process
Next, write a test that executes the main scenario:
public class AuctionTest extends TestCase { // parse the process definition static ProcessDefinition auctionProcess = ProcessDefinition.parseParResource("org/jbpm/tdd/auction.par"); // get the nodes for easy asserting static StartState start = auctionProcess.getStartState(); static State auction = (State) auctionProcess.getNode("auction"); static EndState end = (EndState) auctionProcess.getNode("end"); // the process instance ProcessInstance processInstance; // the main path of execution Token token; public void setUp() { // create a new process instance for the given process definition processInstance = new ProcessInstance(auctionProcess); // the main path of execution is the root token token = processInstance.getRootToken(); } public void testMainScenario() { // after process instance creation, the main path of // execution is positioned in the start state. assertSame(start, token.getNode()); token.signal(); // after the signal, the main path of execution has // moved to the auction state assertSame(auction, token.getNode()); token.signal(); // after the signal, the main path of execution has // moved to the end state and the process has ended assertSame(end, token.getNode()); assertTrue(processInstance.hasEnded()); } }
15.2. XML Sources
Before writing execution scenarios, you must compose a
ProcessDefinition
. The easiest way to obtain a ProcessDefinition
object is by parsing XML. With code completion switched on, type ProcessDefinition.parse
. The various parsing methods will be displayed. There are three ways in which to write XML that can be parsed to a ProcessDefinition
object:
15.2.1. Parsing a Process Archive
A process archive is a ZIP file that contains the process XML file, namely
processdefinition.xml
. The jBPM Process Designer plug-in reads and writes process archives.
static ProcessDefinition auctionProcess = ProcessDefinition.parseParResource("org/jbpm/tdd/auction.par");
15.2.2. Parsing an XML File
To write the
processdefinition.xml
file by hand, use the JpdlXmlReader
. Use an ant
script to package the resulting ZIP file.
static ProcessDefinition auctionProcess = ProcessDefinition.parseXmlResource("org/jbpm/tdd/auction.xml");
15.2.3. Parsing an XML String
Parse the XML in the unit test inline from a plain string:
static ProcessDefinition auctionProcess = ProcessDefinition.parseXmlString( "<process-definition>" + " <start-state name='start'>" + " <transition to='auction'/>" + " </start-state>" + " <state name='auction'>" + " <transition to='end'/>" + " </state>" + " <end-state name='end'/>" + "</process-definition>");